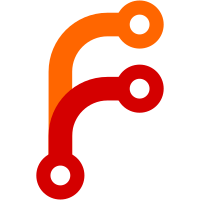
* [MM-39852] Setup docker image to run in CI for E2E * Setup remote docker * Install docker * Trying this * And this * how about this * this * Okay this * dis one * sdfsagsdags * Now? * aaaaaaa * asdasdasd * i am dumb * blank * Please work * Lint fix * Forgot to update a couple things * OOPS * Testing something since this one is still failing * Trying robotjs instead * test * Remove stop docker * Try without the admin user (since apparently turning off admin notices didn't work) * Remove console statement Co-authored-by: Mattermod <mattermod@users.noreply.github.com>
82 lines
3 KiB
JavaScript
82 lines
3 KiB
JavaScript
// Copyright (c) 2016-present Mattermost, Inc. All Rights Reserved.
|
|
// See LICENSE.txt for license information.
|
|
|
|
'use strict';
|
|
|
|
const fs = require('fs');
|
|
|
|
const env = require('../../modules/environment');
|
|
const {asyncSleep} = require('../../modules/utils');
|
|
|
|
describe('RemoveServerModal', function desc() {
|
|
this.timeout(30000);
|
|
const config = env.demoConfig;
|
|
|
|
beforeEach(async () => {
|
|
env.createTestUserDataDir();
|
|
env.cleanTestConfig();
|
|
fs.writeFileSync(env.configFilePath, JSON.stringify(config));
|
|
await asyncSleep(1000);
|
|
this.app = await env.getApp();
|
|
|
|
const mainView = this.app.windows().find((window) => window.url().includes('index'));
|
|
const dropdownView = this.app.windows().find((window) => window.url().includes('dropdown'));
|
|
await mainView.click('.TeamDropdownButton');
|
|
await dropdownView.hover('.TeamDropdown .TeamDropdown__button:nth-child(1)');
|
|
await dropdownView.click('.TeamDropdown .TeamDropdown__button:nth-child(1) button.TeamDropdown__button-remove');
|
|
|
|
removeServerView = await this.app.waitForEvent('window', {
|
|
predicate: (window) => window.url().includes('removeServer'),
|
|
});
|
|
|
|
// wait for autofocus to finish
|
|
await asyncSleep(500);
|
|
});
|
|
|
|
afterEach(async () => {
|
|
if (this.app) {
|
|
await this.app.close();
|
|
}
|
|
});
|
|
|
|
let removeServerView;
|
|
|
|
it('MM-T4390_1 should remove existing team on click Remove', async () => {
|
|
await removeServerView.click('button:has-text("Remove")');
|
|
await asyncSleep(1000);
|
|
|
|
const expectedConfig = JSON.parse(JSON.stringify(config.teams.slice(1)));
|
|
expectedConfig.forEach((value) => {
|
|
value.order--;
|
|
});
|
|
|
|
const savedConfig = JSON.parse(fs.readFileSync(env.configFilePath, 'utf8'));
|
|
savedConfig.teams.should.deep.equal(expectedConfig);
|
|
});
|
|
|
|
it('MM-T4390_2 should NOT remove existing team on click Cancel', async () => {
|
|
await removeServerView.click('button:has-text("Cancel")');
|
|
await asyncSleep(1000);
|
|
|
|
const savedConfig = JSON.parse(fs.readFileSync(env.configFilePath, 'utf8'));
|
|
savedConfig.teams.should.deep.equal(config.teams);
|
|
});
|
|
|
|
it('MM-T4390_3 should disappear on click Close', async () => {
|
|
await removeServerView.click('button.close');
|
|
await asyncSleep(1000);
|
|
const existing = Boolean(await this.app.windows().find((window) => window.url().includes('removeServer')));
|
|
existing.should.be.false;
|
|
});
|
|
|
|
it('MM-T4390_4 should disappear on click background', async () => {
|
|
// ignore any target closed error
|
|
try {
|
|
await removeServerView.click('.modal', {position: {x: 20, y: 20}});
|
|
} catch {} // eslint-disable-line no-empty
|
|
await asyncSleep(1000);
|
|
const existing = Boolean(await this.app.windows().find((window) => window.url().includes('removeServer')));
|
|
existing.should.be.false;
|
|
});
|
|
});
|