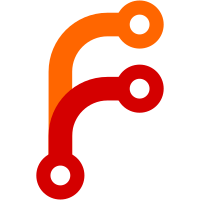
* wip * background download * various fixes * wip * wokring autoupgrade * fix menu * fix windows * cleanup * add publishername * fix messages and titles * Test updates * Moved module and added functionality to click icon to install (instead of just download) * Add auto update setting, update on close app if downloaded * Tests, changes for security fixes, update version number * Update E2E tests * Lint fix * Update to latest electron-updater * Revert to stable electron-builder (only needed to update electron-updater) * Fix package-lock * skip flaky test * Update package * Fix E2E test * Fixes for enabling/disabled autoupdater * Fixed GPO definitions * [MM-38300] Set localhost as the test server * blank * Switch to s3 bucket for testing * Update icons to match spec * Add menu items for download/update actions * Type and test fixes * Fix notification circle * Fix macOS app not restarting on Restart/Update * Update dialog box titles * Turn off file system check for Linux * Changes to support deployments * Testing autoupdater deployments to s3 * disable tests for now * asfrehwf * fine no windows WHATEVER * remove windows again * Try universal all in one * pffftttngggguhhhh * make sure it's working * Missed artifacts script * Modify destination as well * one more time! * Update yml files * Oops * add yq manually * oof * Fix the script to work properly * Fix release script * Fix script again so it runs in time * Build version 2 * Revert build specific changes * Lint override * Fix build apps for PR builds * One more change * Add file generation for .deb repo * Deb repo test * skip tests for now * Fix artifact push * Persist after repo creation * Put tests back * Fix unit tests * Enable mac generated builds temp * Temporarily disable tests * Fix issue where notification doesn't pop dialog box * Try version 2 again * Put the version back * Attempting to debug mac app path issue * Fix issue where Mac app will quarantine itself after first update * Lock versions of yq * Fix yq for mac * As usual, Mac is difficult :P * Add quotes to anti-quarantine command * Change to spawn to avoid command injection * Oops * Nightly deployment changes (#2005) * Test nightly deploy * I fixed a some things * aaaaaaaaa * Restore old bucket * Added progress indicator via tooltip * Ship nightly builds to main S3 bucket * PR feedback * Fix a couple security exploits * Fix opacity on light mode button * Use large app icon * Resize icon for Windows * Resize icon for Mac * Update to electron-updater final * Remove Mac support and deb repo * Typo * Remove deb script * Remove checksum function * Removed autoUpdateSettingsPath * Update URL Co-authored-by: = <=> Co-authored-by: Devin Binnie <devin.binnie@mattermost.com> Co-authored-by: Mattermod <mattermod@users.noreply.github.com> Co-authored-by: Devin Binnie <52460000+devinbinnie@users.noreply.github.com>
104 lines
3.7 KiB
JavaScript
104 lines
3.7 KiB
JavaScript
// Copyright (c) 2016-present Mattermost, Inc. All Rights Reserved.
|
|
// See LICENSE.txt for license information.
|
|
'use strict';
|
|
|
|
const fs = require('fs');
|
|
|
|
const robot = require('robotjs');
|
|
|
|
const env = require('../../modules/environment');
|
|
const {asyncSleep} = require('../../modules/utils');
|
|
|
|
describe('file_menu/dropdown', function desc() {
|
|
this.timeout(30000);
|
|
|
|
const config = env.demoConfig;
|
|
|
|
let skipAfterEach = false;
|
|
|
|
beforeEach(async () => {
|
|
env.createTestUserDataDir();
|
|
env.cleanTestConfig();
|
|
fs.writeFileSync(env.configFilePath, JSON.stringify(config));
|
|
await asyncSleep(1000);
|
|
this.app = await env.getApp();
|
|
|
|
skipAfterEach = false;
|
|
});
|
|
|
|
afterEach(async () => {
|
|
if (this.app && skipAfterEach === false) {
|
|
await this.app.close();
|
|
}
|
|
});
|
|
|
|
it('MM-T1313 Open Settings modal using keyboard shortcuts', async () => {
|
|
const mainWindow = this.app.windows().find((window) => window.url().includes('index'));
|
|
mainWindow.should.not.be.null;
|
|
if (process.platform === 'win32' || process.platform === 'linux') {
|
|
robot.keyTap(',', ['control']);
|
|
const settingsWindow = await this.app.waitForEvent('window', {
|
|
predicate: (window) => window.url().includes('settings'),
|
|
});
|
|
settingsWindow.should.not.be.null;
|
|
}
|
|
});
|
|
|
|
it('MM-T805 Sign in to Another Server Window opens using menu item', async () => {
|
|
if (process.platform === 'win32' || process.platform === 'linux') {
|
|
const mainWindow = this.app.windows().find((window) => window.url().includes('index'));
|
|
mainWindow.should.not.be.null;
|
|
await mainWindow.click('button.three-dot-menu');
|
|
robot.keyTap('f');
|
|
robot.keyTap('s');
|
|
robot.keyTap('s');
|
|
robot.keyTap('enter');
|
|
const signInToAnotherServerWindow = await this.app.waitForEvent('window', {
|
|
predicate: (window) => window.url().includes('newServer'),
|
|
});
|
|
signInToAnotherServerWindow.should.not.be.null;
|
|
}
|
|
});
|
|
|
|
it('MM-T804 Preferences in Menu Bar open the Settings page', async () => {
|
|
if (process.platform !== 'darwin') {
|
|
const mainWindow = this.app.windows().find((window) => window.url().includes('index'));
|
|
mainWindow.should.not.be.null;
|
|
robot.keyTap(',', ['control']);
|
|
const settingsWindow = await this.app.waitForEvent('window', {
|
|
predicate: (window) => window.url().includes('settings'),
|
|
});
|
|
settingsWindow.should.not.be.null;
|
|
robot.keyTap('w', ['control']);
|
|
|
|
//Opening the menu bar
|
|
robot.keyTap('alt');
|
|
robot.keyTap('enter');
|
|
robot.keyTap('f');
|
|
robot.keyTap('s');
|
|
robot.keyTap('enter');
|
|
const settingsWindowFromMenu = await this.app.waitForEvent('window', {
|
|
predicate: (window) => window.url().includes('settings'),
|
|
});
|
|
settingsWindowFromMenu.should.not.be.null;
|
|
}
|
|
});
|
|
|
|
it('MM-T806 Exit in the Menu Bar', () => {
|
|
const mainWindow = this.app.windows().find((window) => window.url().includes('index'));
|
|
mainWindow.should.not.be.null;
|
|
|
|
if (process.platform === 'darwin') {
|
|
robot.keyTap('q', ['command']);
|
|
}
|
|
|
|
if (process.platform === 'linux' || process.platform === 'win32') {
|
|
robot.keyTap('q', ['control']);
|
|
}
|
|
|
|
this.app.windows().find((window) => window.url().should.not.include('index'));
|
|
|
|
skipAfterEach = true; // Need to skip closing in aftereach as apps execution context is destroyed above
|
|
});
|
|
});
|